Full Adder is a combinational logic circuit that adds three inputs and produces two outputs. The diagram below illustrates the basic block diagram and circuit diagram of a Full Adder, where A, B, and C_in are the inputs, and Sum and C_out are the outputs. Here, C_in is commonly referred to as the carry input, and C_out is known as the carry output.
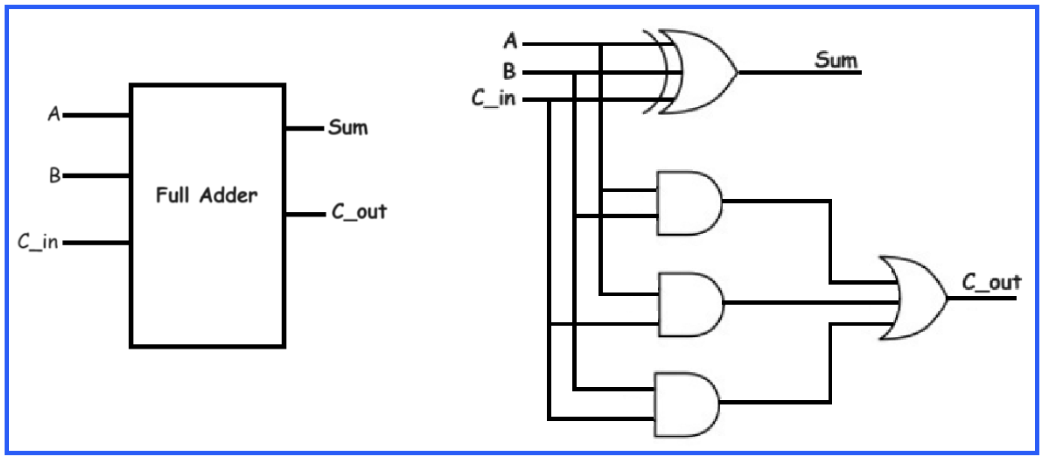
The table below presents the truth table of a Full Adder.
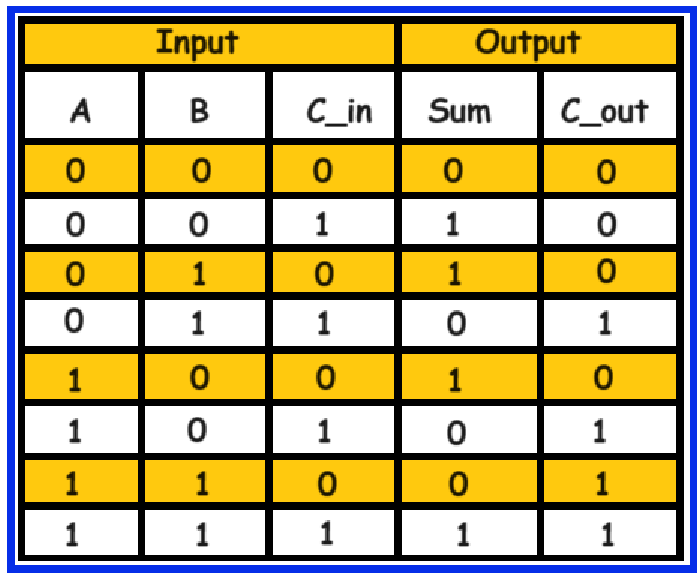
From the input, we can observe that the sum output is high only when at least one input is equal to 1 or when all inputs are equal to 1. C_out is equal to 1 when two or three inputs are equal to 1. The output equations of a Full Adder are as follows:
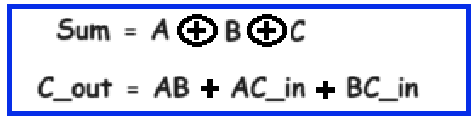
If we want to design the Full Adder using half adders, the circuit diagram and block diagram for it will be as follows:
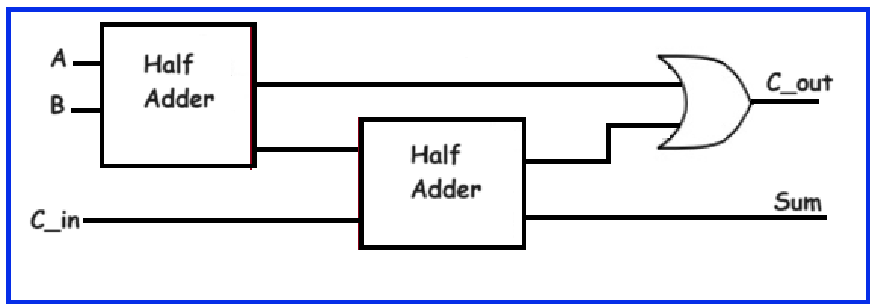
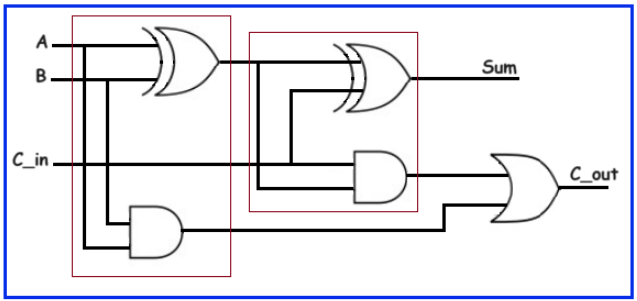
Therefore, we need two Half Adders and one OR gate to design a Full Adder.
In this project, we will design and implement the Full Adder using Half Adders with the Xilinx Vivado design tool.
Step 1: Click on New Project -> Next
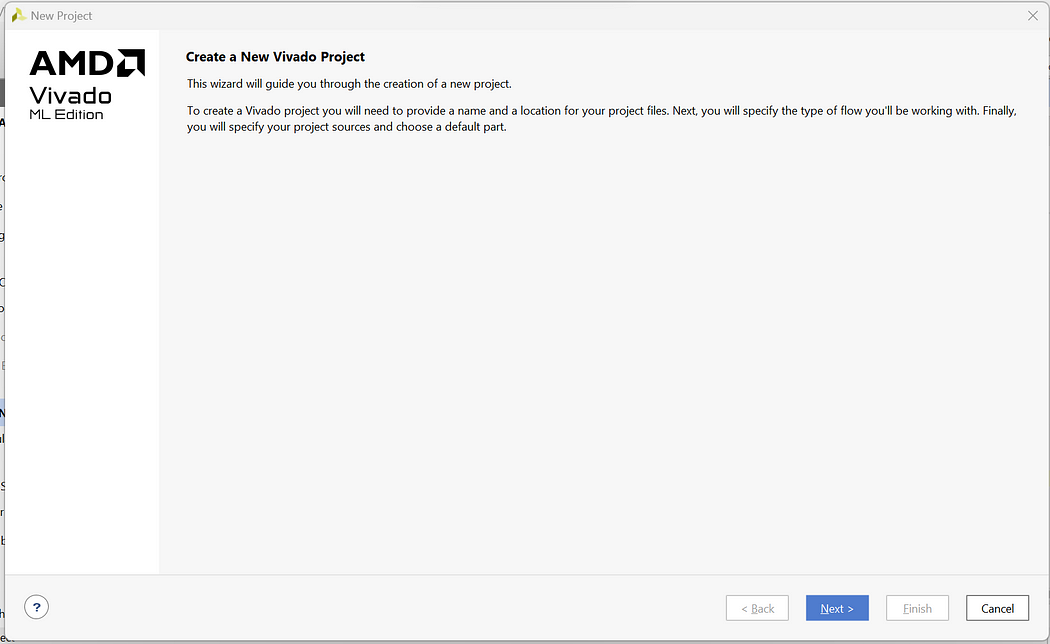
Step 2: Enter Project Name and Select appropriate Project Location -> Next
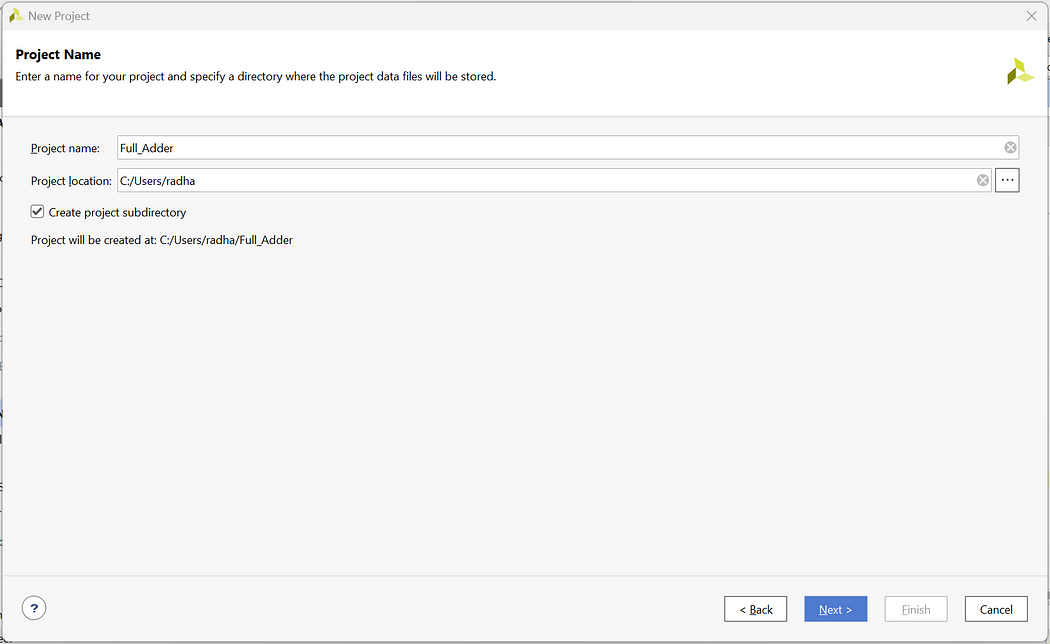
Step 3: Select RTL Project -> Next
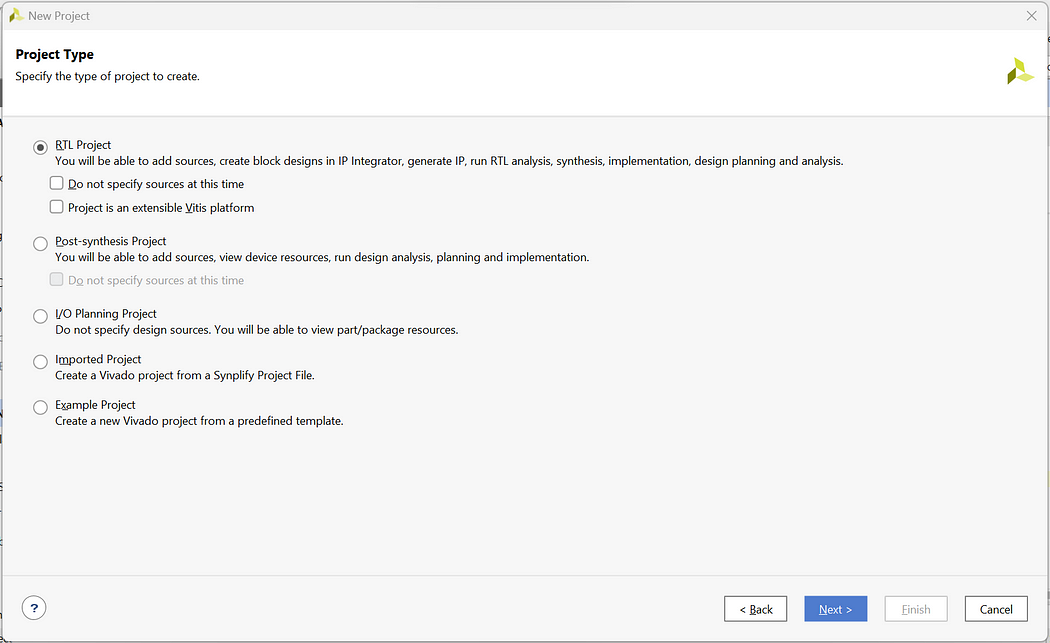
Step 4: Click on Create File and create 3 files with file names or_gate, half_adder and full_adder -> Next
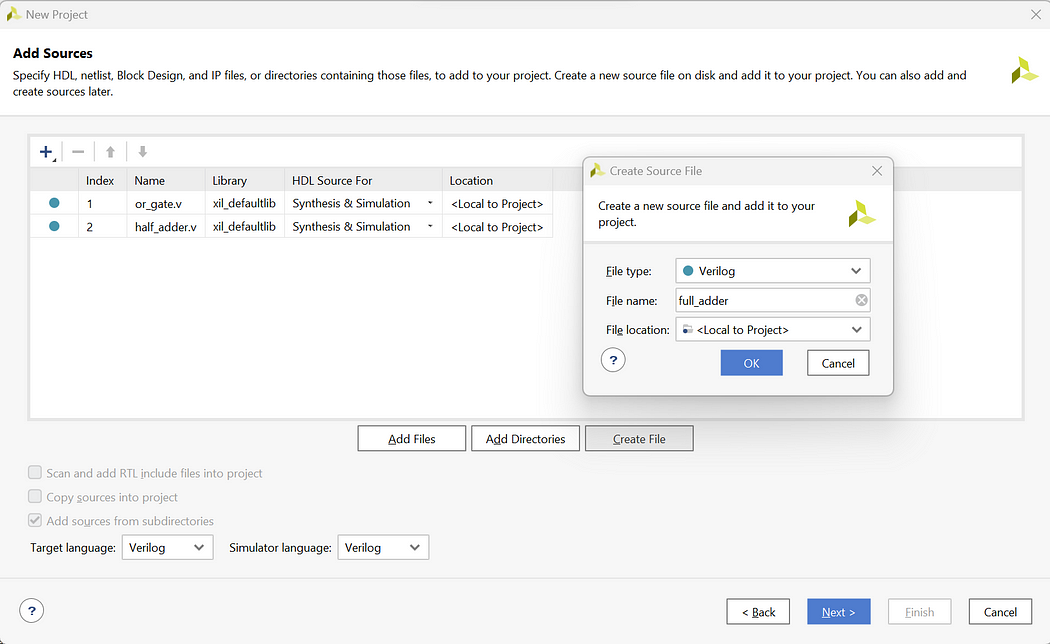
Step 5: We will not add the Constraint file So click on Next
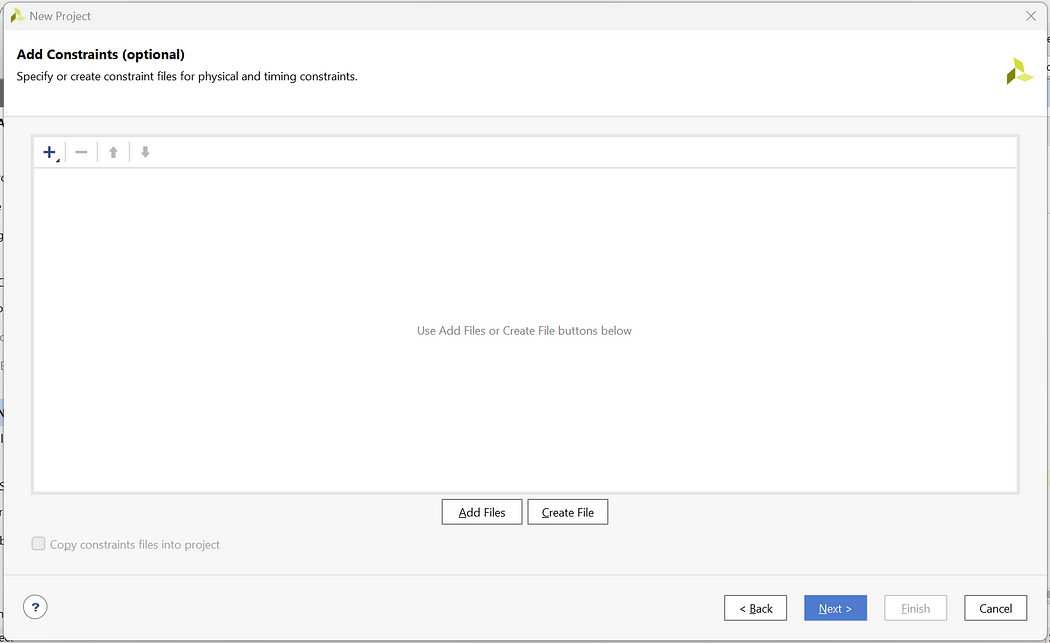
Step 6: For Default Part click on Next as we are not using any development Board
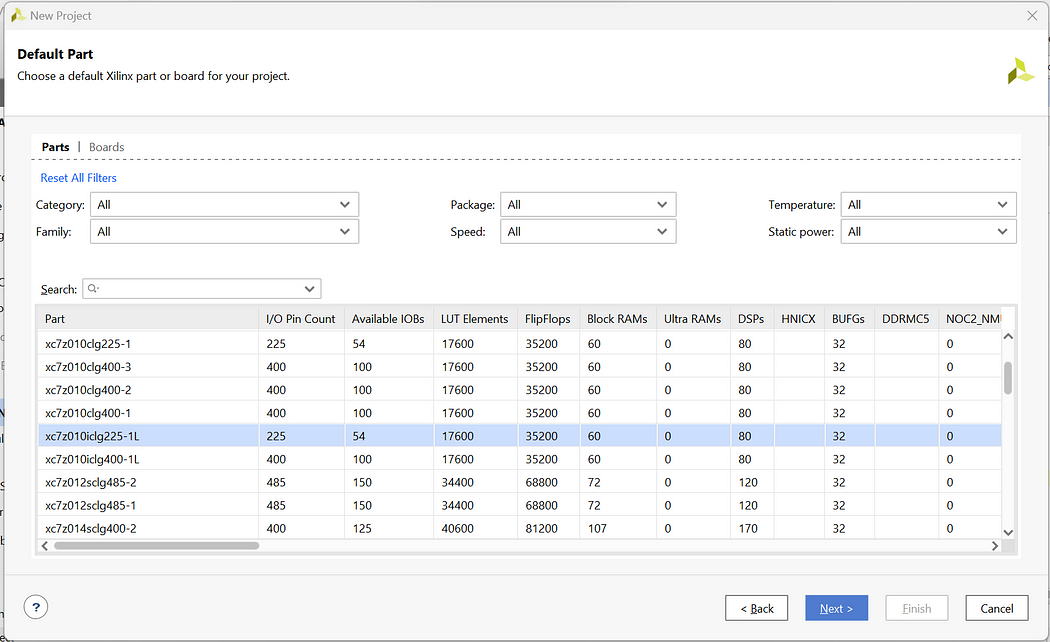
Step 7: Check the New Project Summary and click on Finish
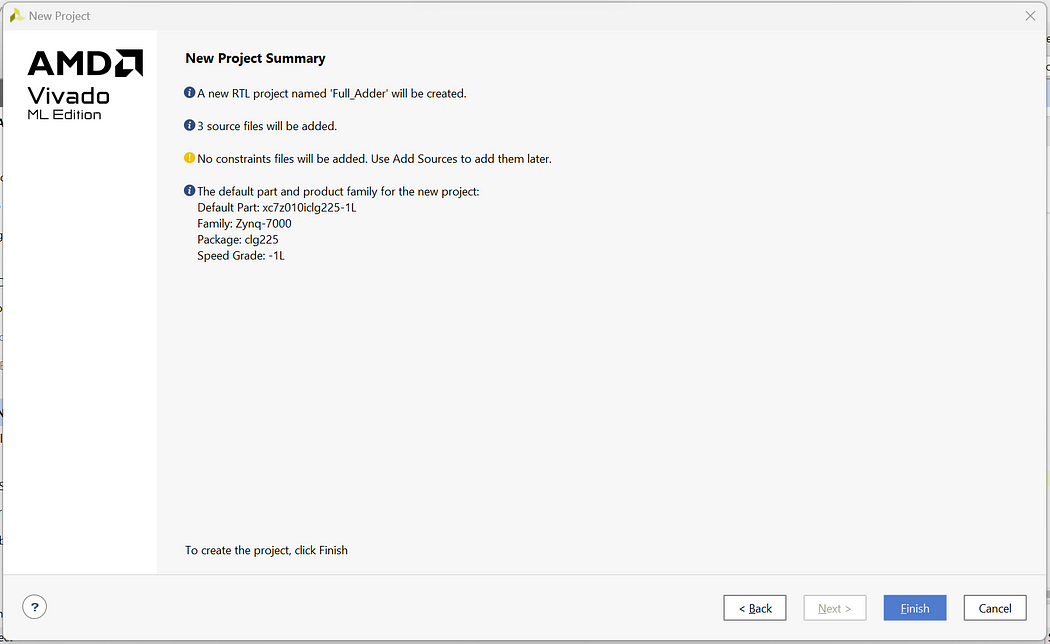
Step 8: Now you will be prompted with the Define module page but we will create the complete code from scratch so click on cancel and Yes
Step 9: Now on the Home Page click on Sources -> Design Sources -> Non-module Files
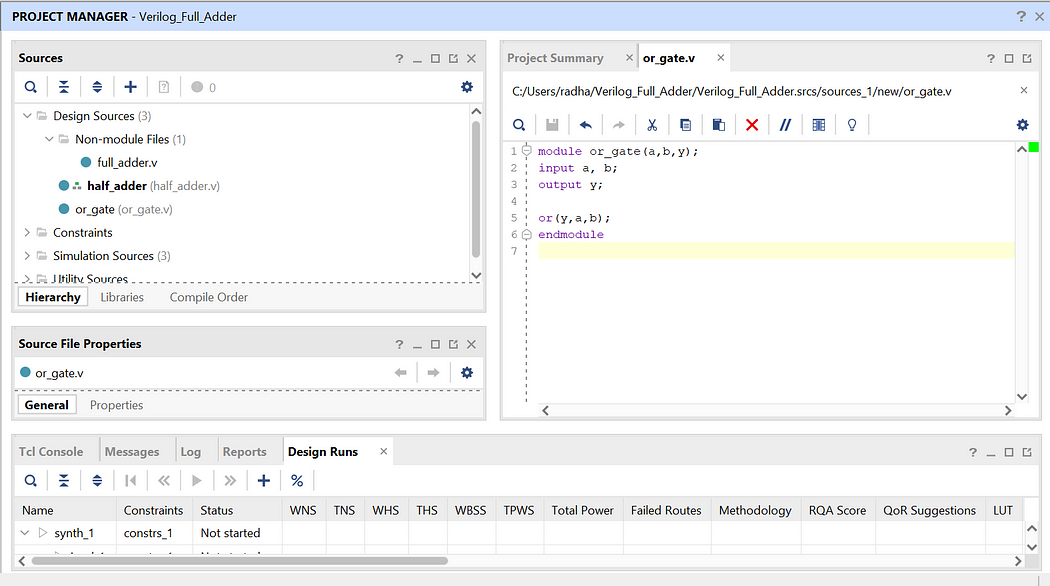
Step 10: Enter the below codes in the “.v” files
or_gate.v
module or_gate(a,b,y);
input a, b;
output y;
or(y,a,b);
endmodule
half_adder.v
module half_adder(a,b,sum,carry);
input a, b;
output sum, carry;
xor(sum,a,b);
and(carry,a,b);
endmodule
full_adder.v
module full_adder(a,b,cin,sum,carry);
input a,b, cin;
output sum, carry;
wire c1,c2,s1;
half_adder uut1 (a,b,s1,c1);
half_adder uut2 (cin,s1,sum,c2);
or_gate uut3(c1,c2,carry);
endmodule
Here, we have written verilog code for or gate, half adder and full adder.
Step 11: Now to write the testbench code for above design right click on Design Sources -> Add Sources -> Add or create design sources -> Create File -> Add File name as tb_full_adder -> Finish -> Ok -> Yes
Step 12: Now open the testbench file and enter the below testbench code
module tb_full_adder;
reg a;
reg b;
reg cin;
wire sum;
wire carry;
full_adder UUT (.a(a), .b(b),.cin(cin), .sum(sum), .carry(carry));
initial begin
$display(“Testing Full Adder gate”);
a = 0; b=0; cin=0;
#10;
$display(“Input_A = %b, Input_B = %b, Input_Cin = %b, Sum = %b, Carry = %b” , a,b,cin,sum,carry);
a = 0; b=0; cin=1;
#10;
$display(“Input_A = %b, Input_B = %b, Input_Cin = %b, Sum = %b, Carry = %b” , a,b,cin,sum,carry);
a = 0; b=1; cin=0;
#10;
$display(“Input_A = %b, Input_B = %b, Input_Cin = %b, Sum = %b, Carry = %b” , a,b,cin,sum,carry);
a = 0; b=1; cin=1;
#10;
$display(“Input_A = %b, Input_B = %b, Input_Cin = %b, Sum = %b, Carry = %b” , a,b,cin,sum,carry);
a = 1; b=0; cin=0;
#10;
$display(“Input_A = %b, Input_B = %b, Input_Cin = %b, Sum = %b, Carry = %b” , a,b,cin,sum,carry);
a = 1; b=0; cin=1;
#10;
$display(“Input_A = %b, Input_B = %b, Input_Cin = %b, Sum = %b, Carry = %b” , a,b,cin,sum,carry);
a = 1; b=1; cin=0;
#10;
$display(“Input_A = %b, Input_B = %b, Input_Cin = %b, Sum = %b, Carry = %b” , a,b,cin,sum,carry);
a = 1; b=1; cin=1;
#10;
$display(“Input_A = %b, Input_B = %b, Input_Cin = %b, Sum = %b, Carry = %b” , a,b,cin,sum,carry);
$finish;
end
endmodule
Here, in testbench code we do not define values in entity hence we have kept it empty. Then in architecture we have copied the components of full_adder and defined signals for connecting the ports of full adder. Inside the process statement we write all 8 test cases from truth table and define a delay.
Step 13: Now as we have written all the codes let’s launch the simulation. Enter launch_simulation in the Tcl Console and press Enter
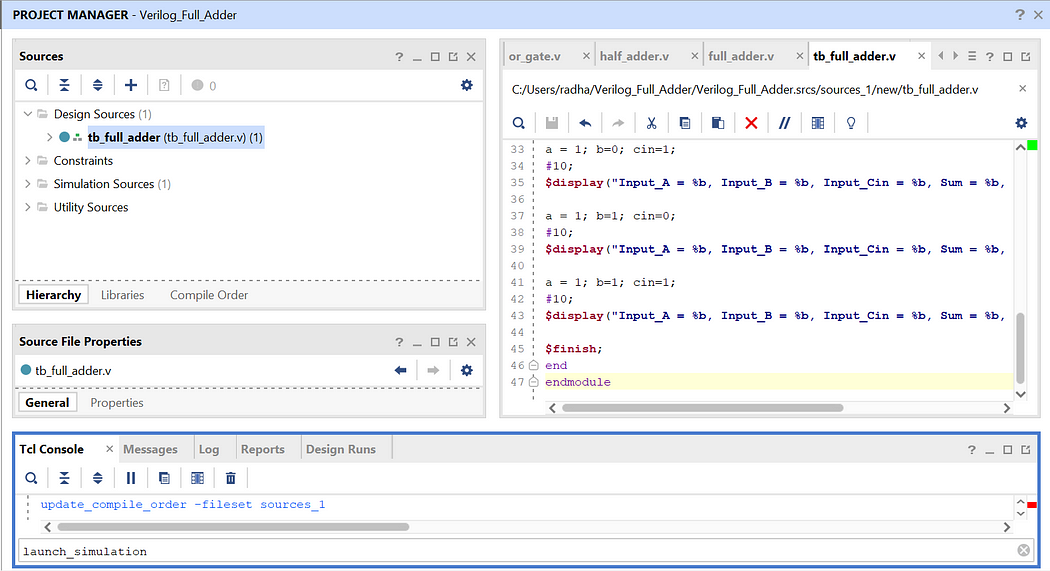
We have successfully implemented full adder using half adder with testbench code. Click on Zoom Fit to see the output waveform more clearly and verify the outputs.
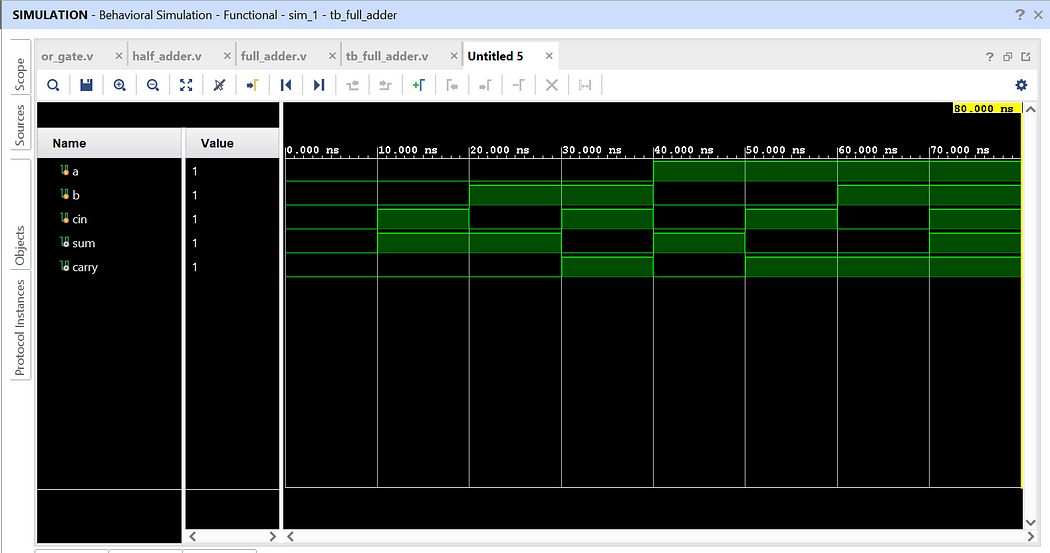