In our ongoing journey to master Verilog, we’ve explored various foundational concepts and advanced features. In this segment, we will focus on two crucial constructs in Verilog programming: Tasks and Functions. Understanding these constructs is essential for creating modular, reusable, and efficient Verilog code.
1. Introduction to Tasks and Functions
Tasks and functions in Verilog are used to encapsulate and reuse code. They help in organizing code into manageable pieces, making it more readable and maintainable. While both tasks and functions serve similar purposes, they have distinct characteristics and use cases.
Often, we encounter repetitive code segments in RTL (Register Transfer Level) that are invoked multiple times. These segments typically do not consume simulation time and often involve complex calculations with varying data values. In such instances, declaring a function to encapsulate the repetitive code can be highly beneficial. A function allows you to process inputs and return a single value, reducing the amount of RTL code you need to write. By calling the function and passing the necessary data for computation, you streamline your code and avoid redundancy.
In contrast, a task is more versatile. Tasks can handle multiple result values, returning them through output or inout arguments. They can include simulation time-consuming elements like ‘@’ or ‘posedge’. While functions do not consume simulation time and return only a single value, tasks may or may not consume simulation time and can return values via output or inout arguments.
Verilog Tasks
A task in Verilog is used to perform a sequence of statements and can include delays and timing control. Tasks are useful for operations that may require multiple statements and potentially involve waiting periods.
Syntax:
task task_name;
// Input and output declarations
input [width-1:0] input_name;
output [width-1:0] output_name;// Task body
begin
// Task operations
end
endtask
Example:
module TaskExample;
reg [7:0] a, b;
reg [7:0] result;
// Task Definition
task add_two_numbers;
input [7:0] num1, num2;
output [7:0] sum;
begin
#5 sum = num1 + num2; // Perform addition with a delay
end
endtaskinitial begin
a = 8'd10;
b = 8'd20;
add_two_numbers(a, b, result); // Call the task
$display(“The result of addition is: %d”, result);
$stop;
end
endmodule
Explanation:
- Task Definition: ‘add_two_numbers’ takes two inputs (‘num1’ and ‘num2’) and provides an output (‘sum’), with a delay of 5 time units before computing the sum.
- Task Call: The task is invoked in the ‘initial’ block to perform the addition.
Key Features of Tasks:
- Can contain delays and timing controls.
- Can have input, output, and inout arguments.
- Can call other tasks.
- May or may not consume simulation time, depending on their contents.
Verilog Functions
A function in Verilog is used for computing a value and returning it. Functions are typically used for simple calculations and must return a single value. They cannot contain delays or timing controls.
Syntax:
function [return_width-1:0] function_name;
input [input_width-1:0] input_name;
// Function body
begin
function_name = expression; // Compute and return value
end
endfunction
Example:
module FunctionExample;
reg [7:0] a, b;
reg [7:0] result;// Function Definition
function [7:0] add_two_numbers;
input [7:0] num1, num2;
begin
add_two_numbers = num1 + num2; // Compute the sum
end
endfunctioninitial begin
a = 8'd15;
b = 8'd25;
result = add_two_numbers(a, b); // Call the function
$display(“The result of addition is: %d”, result);
$stop;
end
endmodule
Explanation:
- Function Definition: ‘add_two_numbers’ takes two inputs and returns their sum.
- Function Call: The function is called in the ‘initial’ block to compute and return the result.
Key Features of Functions:
- Cannot contain delays or timing controls.
- Must return a single value.
- Cannot call tasks.
- Can be used within expressions.
Differences Between Tasks and Functions
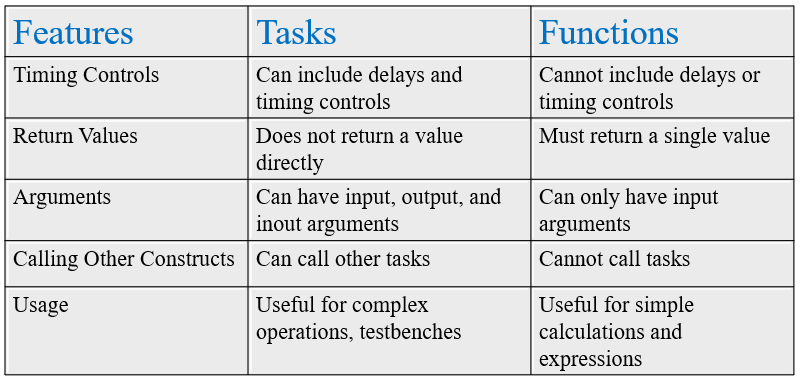
Practical Examples
- Task Example
Suppose you are designing a complex digital system where you need to perform a sequence of operations with delays. A task can be used to encapsulate this logic and improve code readability. - Function Example
For simple calculations such as computing a checksum or performing bitwise operations, functions can be used within expressions to streamline your code.
Conclusion
Tasks and functions are powerful constructs in Verilog that enable modular, reusable, and efficient coding practices. Tasks are suited for complex operations with timing controls, while functions are ideal for simple computations. By encapsulating repetitive code segments in functions and leveraging tasks for more complex operations, you can enhance code maintainability and efficiency. Mastering these constructs will elevate your ability to design and verify digital systems effectively.
Stay tuned for more insights and advanced topics in our mastering Verilog series!