In this project we will see how to implement all Counters with testbench code on Xilinx Vivado design tool.
Step 1: Click on New Project -> Next
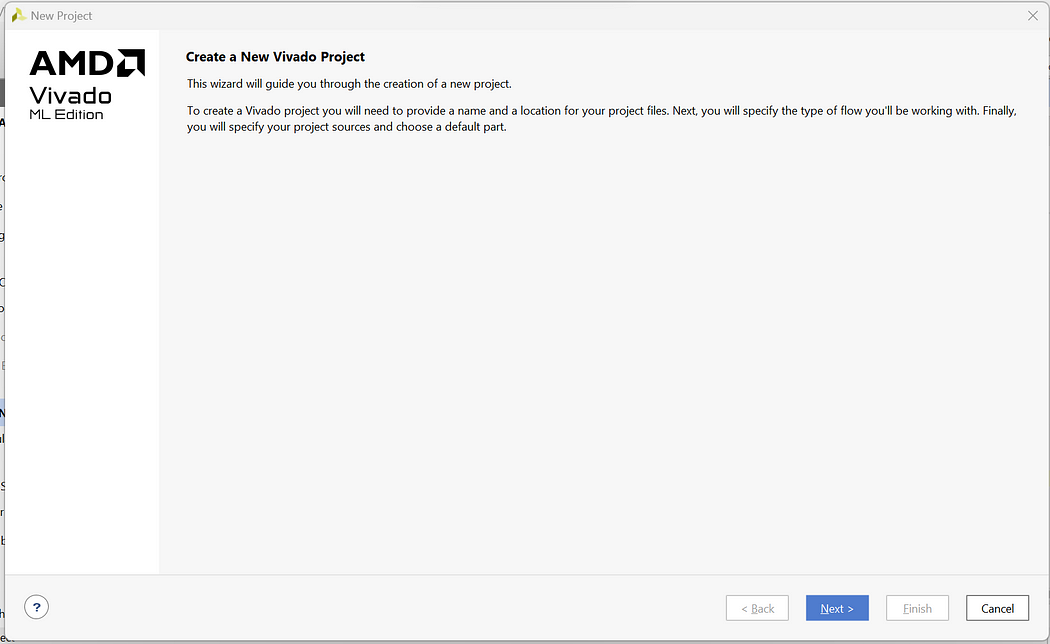
Step 2: Enter Project Name and Select appropriate Project Location -> Next
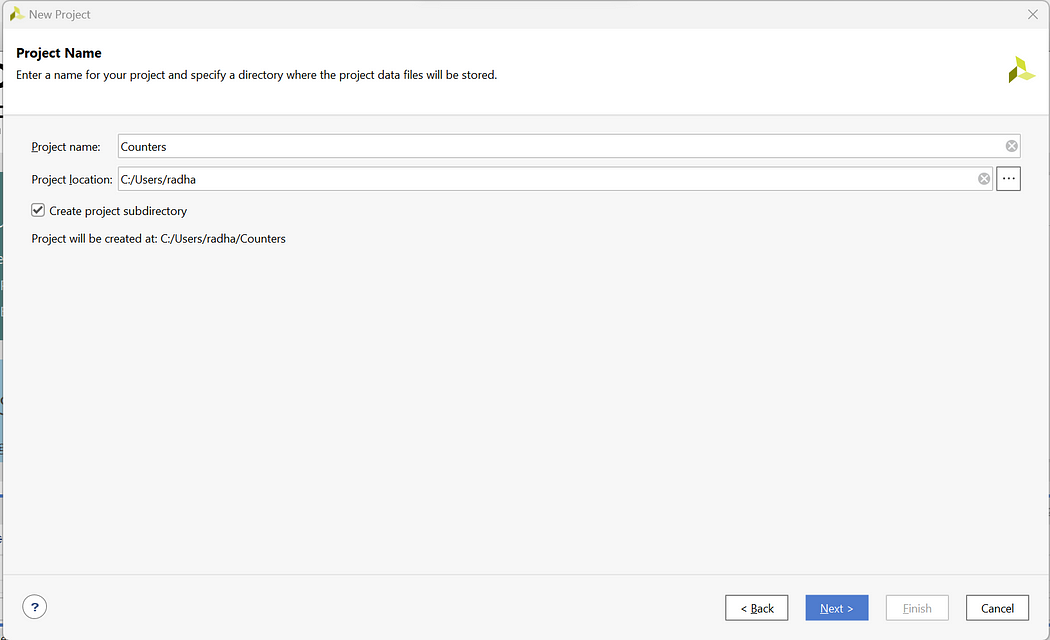
Step 3: Select RTL Project -> Next
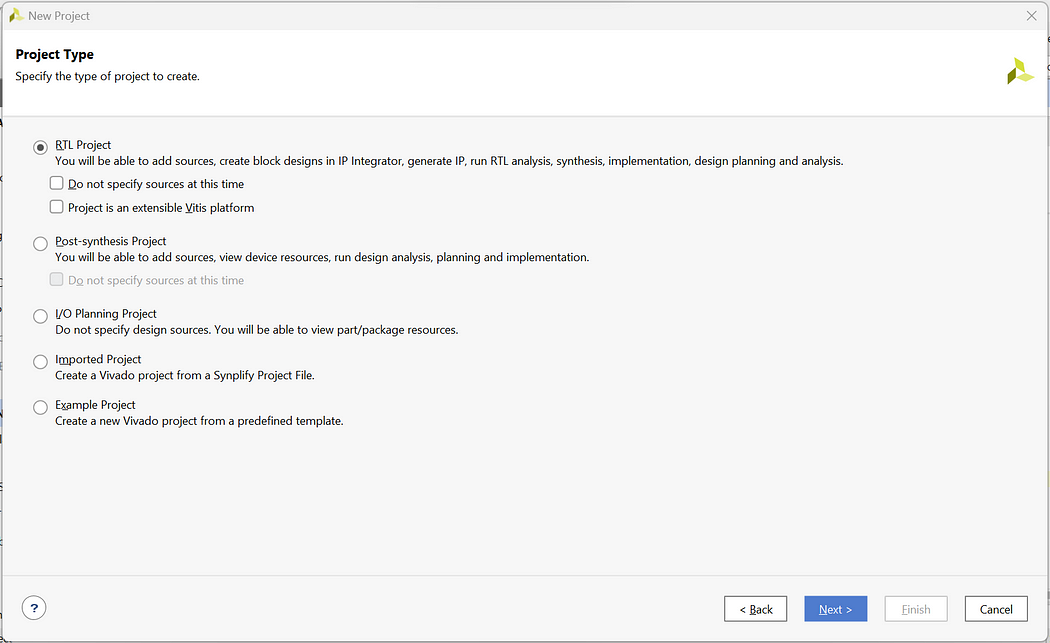
Step 4: Click on Create File and create file with file name up_counter -> Next
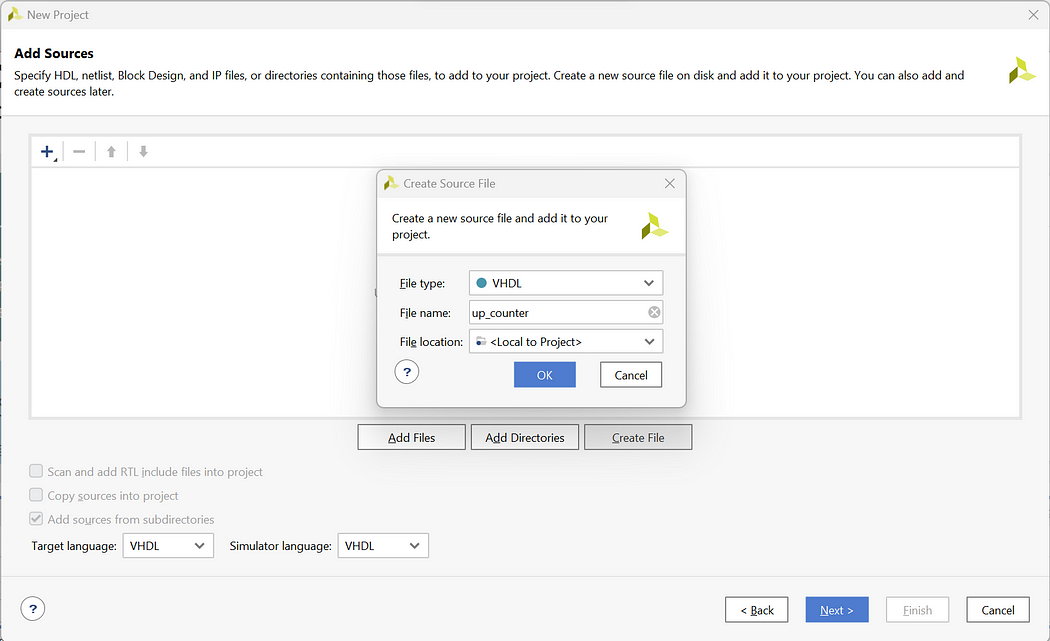
Step 5: We will not add the Constraint file So click on Next
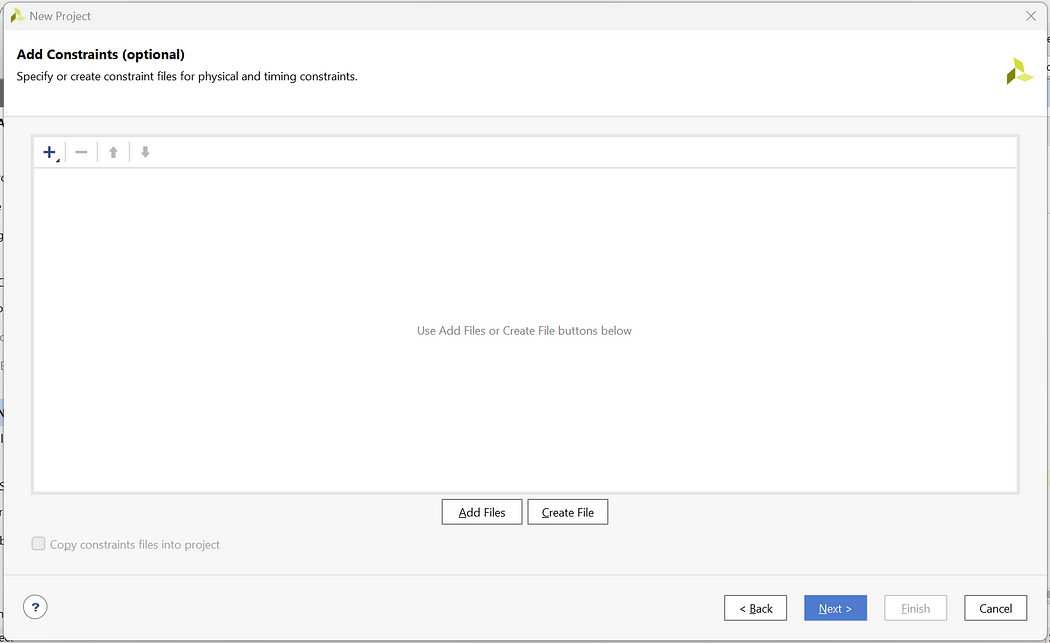
Step 6: For Default Part click on Next as we are not using any development Board
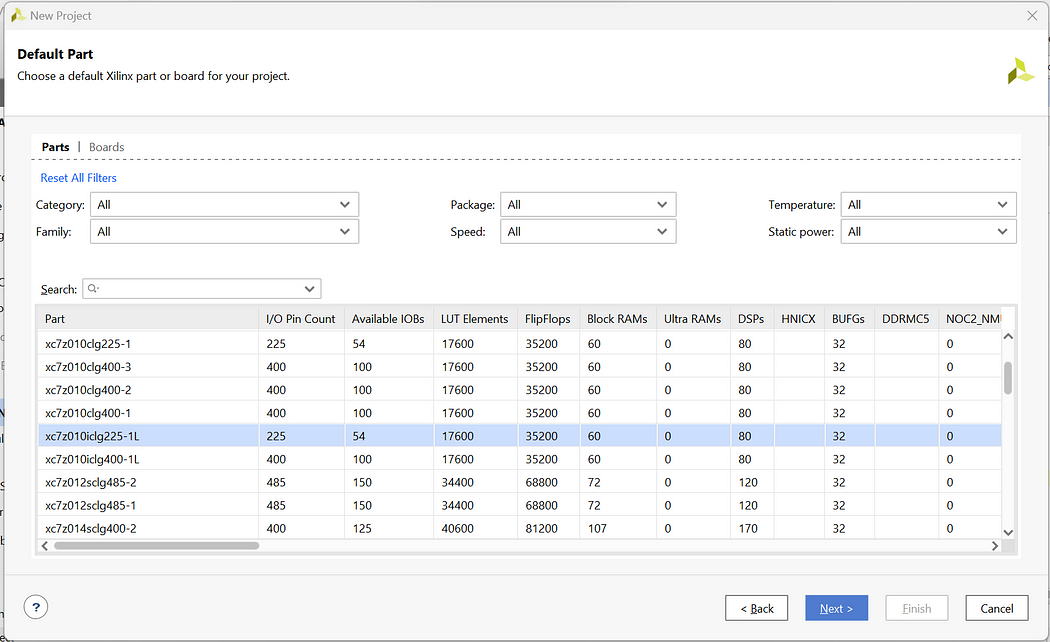
Step 7: Check the New Project Summary and click on Finish.

Step 8: Now you will be prompted with the Define module page but we will create the complete code from scratch so click on cancel and Yes
Step 9: Now on the Home Page click on Sources -> Design Sources -> Non-module Files
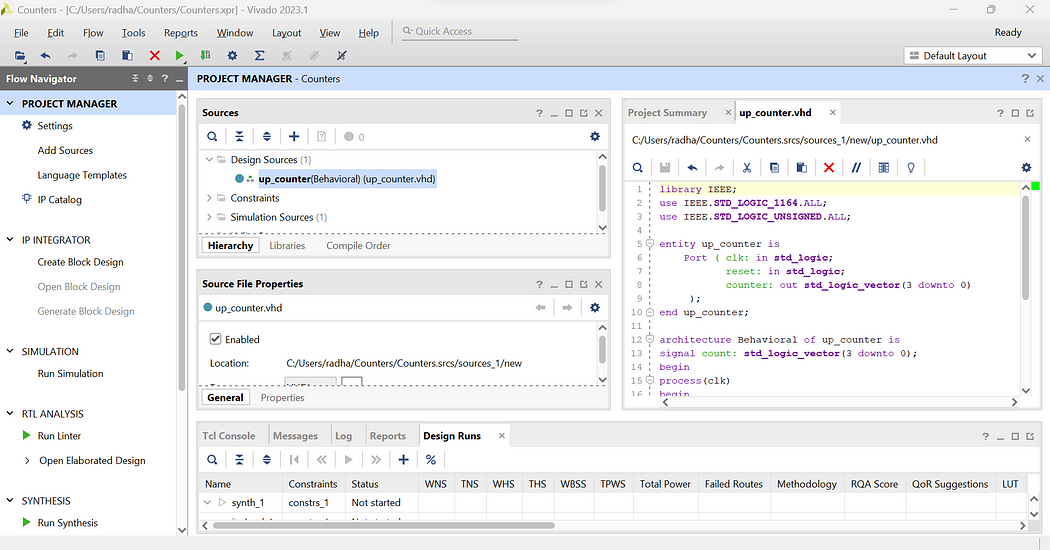
Step 10: Enter the below codes in the “up_counter.vhd” files.
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
entity up_counter is
Port ( clk: in std_logic;
reset: in std_logic;
counter: out std_logic_vector(3 downto 0)
);
end up_counter;
architecture Behavioral of up_counter is
signal count: std_logic_vector(3 downto 0);
begin
process(clk)
begin
if(rising_edge(clk)) then
if(reset=’1') then
count <= x”0";
else
count <= count + x”1";
end if;
end if;
end process;
counter <= count;
end Behavioral;
Step 11: Now to write the testbench code for and gate right click on Design Sources -> Add Sources -> Add or create design sources -> Create File -> Add File name as tb_up_counter -> Finish -> Ok -> Yes
Step 12: Now open the testbench file and enter the below testbench code
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity tb_up_counters is
end tb_up_counters;
architecture Behavioral of tb_up_counters is
component up_counter
Port ( clk: in std_logic; — clock input
reset: in std_logic; — reset input
counter: out std_logic_vector(3 downto 0) — output 4-bit counter
);
end component;
signal reset,clk: std_logic;
signal counter:std_logic_vector(3 downto 0);
begin
dut: up_counter port map (clk => clk, reset=>reset, counter => counter);
— Clock process definitions
clock_process :process
begin
clk <= ‘0’;
wait for 10 ns;
clk <= ‘1’;
wait for 10 ns;
end process;
— Stimulus process
stim_proc: process
begin
— hold reset state for 100 ns.
reset <= ‘1’;
wait for 20 ns;
reset <= ‘0’;
wait;
end process;
end Behavioral;
Here, in testbench code we do not define values in entity hence we have kept it empty. Then in architecture we have copied the components of up_counter and defined signals for connecting the ports of counter. Inside the process statement we write all test cases and define the respective delays.
Step 13: Now as we have written all the codes let’s launch the simulation. Enter launch_simulation in the Tcl Console and press Enter.
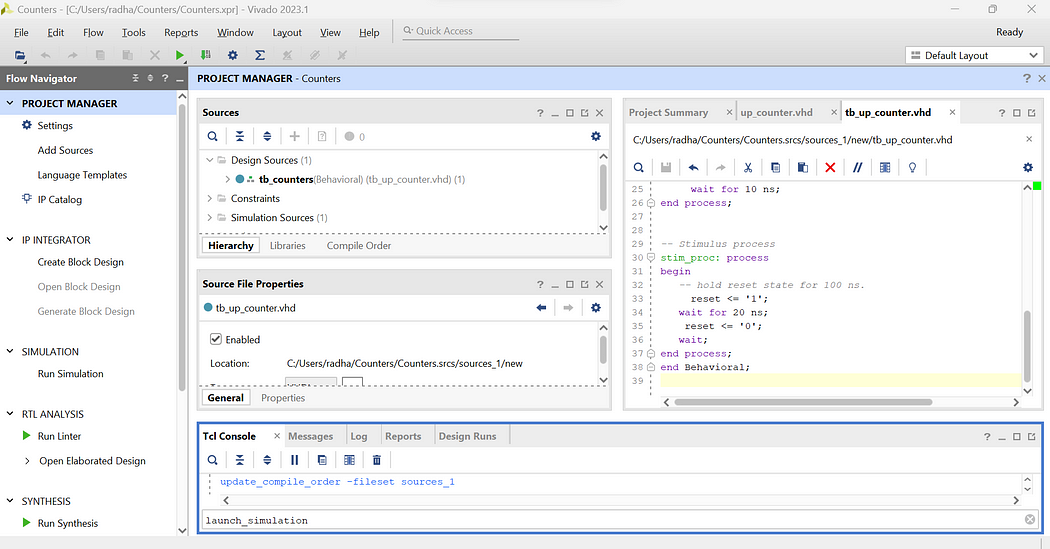
We have successfully implemented up counter with testbench code. Click on Zoom Fit to see the output waveform more clearly and verify the outputs.
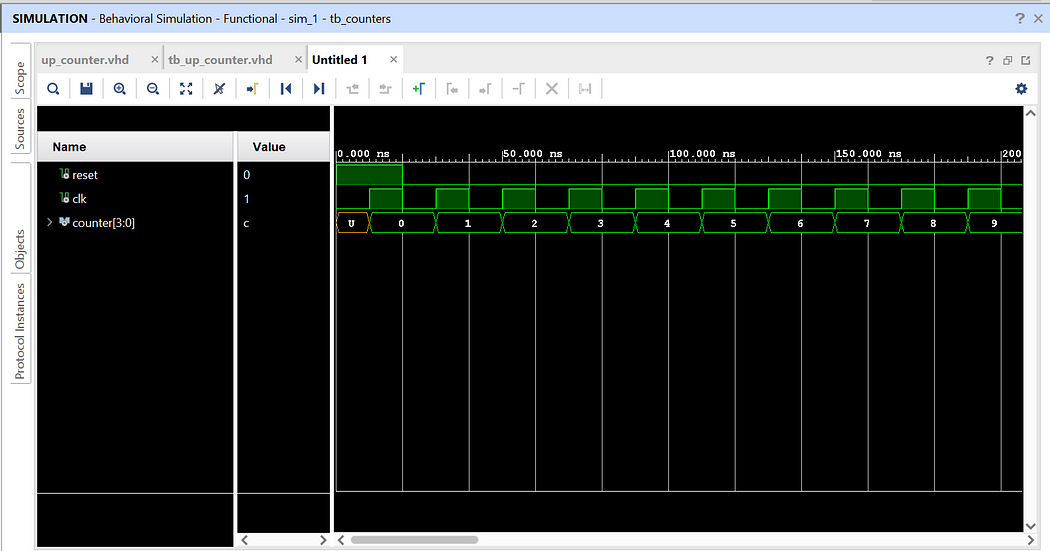
If you want to implement other counters the process would be same except the VHDL codes. Visit below links to see how to implement other Counters:
1] Down Counter
2] Up Down Counter
In this way, we can implement Counters using testbench codes.